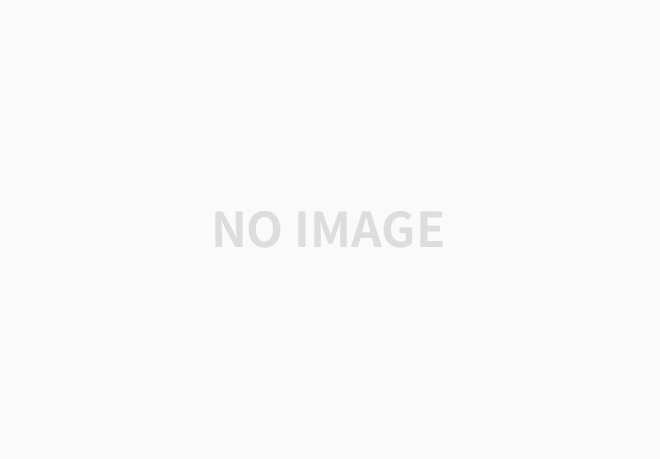
A Docker image is a read-only template that contains everything needed to run a container, including the application, libraries, and configuration files. This guide outlines efficient methods for building and managing Docker images.
1. Building a Docker Image
1.1 Writing a Dockerfile
A Docker image is created based on a Dockerfile.
Here is a basic example:
# Set base image
FROM python:3.9
# Set working directory
WORKDIR /app
# Copy dependencies and install required packages
COPY requirements.txt .
RUN pip install -r requirements.txt
# Copy application files
COPY . .
# Define the command to run the application
CMD ["python", "app.py"]
1.2 Building a Docker Image
To build an image using the above Dockerfile, run:
docker build -t myapp:latest .
- -t myapp:latest โ Assigns the name myapp with the tag latest.
- . โ Uses the Dockerfile in the current directory.
2. Managing Docker Images
2.1 Listing Docker Images
To check available Docker images:
docker images
2.2 Deleting a Docker Image
To remove a specific image:
docker rmi [image-name] # Or use the image ID
2.3 Changing an Image Tag
To tag an existing image with a new repository and version:
docker tag myapp:latest myrepo/myapp:v1.0
To upload an image to Docker Hub, first log in:
docker login
docker push myrepo/myapp:v1.0
For private registries, include the registry address:
docker tag myapp:latest myregistry.com/myapp:v1.0 docker push myregistry.com/myapp:v1.0
3. Optimizing Docker Images
3.1 Using Multi-Stage Builds
To minimize image size, use multi-stage builds:
# Build stage
FROM node:18 AS builder
WORKDIR /app
COPY package.json .
RUN npm install COPY . .
RUN npm run build
# Runtime stage
FROM nginx:alpine
COPY --from=builder /app/build /usr/share/nginx/html
This approach prevents unnecessary files from being included in the final image.
3.2 Excluding Unnecessary Files
Create a .dockerignore file to prevent unwanted files from being copied:
node_modules
*.log
.git
3.3 Using Lightweight Base Images
Using minimal base images reduces overall image size:
- Instead of python, use python:alpine.
- Instead of ubuntu, use debian or alpine.
Summary
Task | Command /Concept |
Build an image | docker build -t myapp:latest . |
List images | docker images |
Remove an image | docker rmi [image-name] |
Tag an image | docker tag myapp:latest myrepo/myapp:v1.0 |
Push to Docker Hub | docker push myrepo/myapp:v1.0 |
Use Multi-Stage Builds | Reduces final image size |
Exclude unnecessary files | Use .dockerignore |
Use lightweight images | Prefer alpine or debian over ubuntu |
Following these best practices ensures efficient Docker image management and optimized container deployments.
'๊ฐ๋ฐ Code > ๋ถ๋ก Supplement' ์นดํ ๊ณ ๋ฆฌ์ ๋ค๋ฅธ ๊ธ
[Supplement][Docker] Understanding Docker (0) | 2025.02.12 |
---|---|
[Supplement][Docker] ๋์ปค ์ด๋ฏธ์ง ๋น๋ ๋ฐ ๊ด๋ฆฌ (0) | 2025.02.09 |
[Supplement][Docker] Docker ๊ฐ๋ ์ ๋ฆฌ (0) | 2025.02.07 |